Here’s a step-by-step guide on How to Create a WordPress Theme
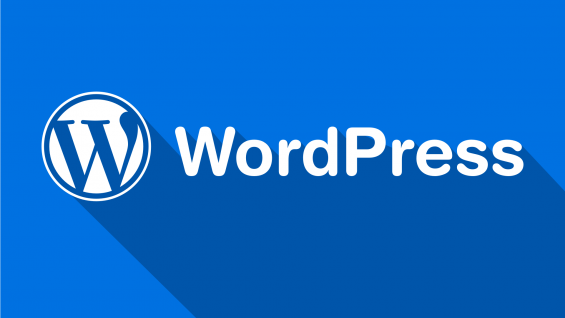
Introduction to WordPress Themes
A WordPress theme determines the layout and design of your website. Creating a custom theme allows you to have full control over how your website looks and functions. In this guide, we will walk you through the process of building a basic WordPress theme from scratch.
1. Set Up a Local Development Environment
Before you start coding your WordPress theme, you need to set up a local development environment. This will allow you to develop and test your theme before launching it on a live site.
- Install a local server environment like XAMPP or Local by Flywheel.
- Install WordPress locally by downloading the WordPress package from WordPress.org and setting it up on your local server.
2. Create a Theme Folder
The next step is to create a new folder for your theme.
- Navigate to the
wp-content/themes
directory in your local WordPress installation. - Create a new folder and name it something unique, for example,
my-custom-theme
.
3. Create the style.css
File
The style.css
file is essential for every WordPress theme. It contains the information about your theme (name, description, version, etc.) and your custom styles.
- Inside your theme folder, create a file named
style.css
. - Add the following header information to the top of the file:
/*
Theme Name: My Custom Theme
Theme URI: http://example.com/my-custom-theme
Author: Your Name
Author URI: http://example.com
Description: A custom WordPress theme built from scratch.
Version: 1.0
License: GNU General Public License v2 or later
License URI: http://www.gnu.org/licenses/gpl-2.0.html
Text Domain: my-custom-theme
*/
You can customize the fields above as needed.
4. Create the index.php
File
The index.php
file is the main template file of your theme. WordPress will use this file to display content if no other templates are available.
- Inside your theme folder, create a file named
index.php
. - Add a basic HTML structure to it:
<!DOCTYPE html>
<html <?php language_attributes(); ?>>
<head>
<meta charset="<?php bloginfo( 'charset' ); ?>">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title><?php bloginfo( 'name' ); ?> - <?php bloginfo( 'description' ); ?></title>
<?php wp_head(); ?>
</head>
<body <?php body_class(); ?>>
<header>
<h1><?php bloginfo( 'name' ); ?></h1>
<nav>
<?php wp_nav_menu( array( 'theme_location' => 'main_menu' ) ); ?>
</nav>
</header>
<main>
<h2>Welcome to My Custom Theme</h2>
<p>This is the homepage of your new WordPress theme.</p>
</main>
<?php wp_footer(); ?>
</body>
</html>
5. Add the functions.php
File
The functions.php
file is used to add custom functionality to your theme. It’s also where you’ll enqueue styles and scripts.
- Inside your theme folder, create a file named
functions.php
. - Add the following code to load the necessary styles and scripts:
<?php
function my_custom_theme_scripts() {
wp_enqueue_style( 'style', get_stylesheet_uri() );
}
add_action( 'wp_enqueue_scripts', 'my_custom_theme_scripts' );
?>
This code ensures that your style.css
file is loaded on your site.
6. Create a Basic Header and Footer
A good practice is to separate your header and footer into individual template files. This makes your theme easier to manage.
- Create a file named
header.php
and add the following code:
<!DOCTYPE html>
<html <?php language_attributes(); ?>>
<head>
<meta charset="<?php bloginfo( 'charset' ); ?>">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title><?php bloginfo( 'name' ); ?> - <?php bloginfo( 'description' ); ?></title>
<?php wp_head(); ?>
</head>
<body <?php body_class(); ?>>
<header>
<h1><?php bloginfo( 'name' ); ?></h1>
<nav>
<?php wp_nav_menu( array( 'theme_location' => 'main_menu' ) ); ?>
</nav>
</header>
- Create a file named
footer.php
and add this code:
<footer>
<p>© <?php echo date( 'Y' ); ?> My Custom Theme. All rights reserved.</p>
</footer>
<?php wp_footer(); ?>
</body>
</html>
- In your
index.php
, replace the header and footer sections with:
<?php get_header(); ?>
<main>
<h2>Welcome to My Custom Theme</h2>
<p>This is the homepage of your new WordPress theme.</p>
</main>
<?php get_footer(); ?>
7. Register a Navigation Menu
To make your theme functional, you need to register a navigation menu so that you can add a menu through the WordPress admin.
- Add this code to your
functions.php
:
function my_custom_theme_setup() {
register_nav_menus( array(
'main_menu' => 'Main Navigation Menu',
) );
}
add_action( 'after_setup_theme', 'my_custom_theme_setup' );
8. Activate Your Theme
Once you’ve created all the necessary files, it’s time to activate your theme.
- Go to the WordPress admin panel.
- Navigate to Appearance > Themes.
- Find your theme and click Activate.
9. Customize and Extend Your Theme
At this point, you’ve created a basic WordPress theme. However, WordPress themes can be much more complex. You can further customize your theme by adding:
- Template files (like
single.php
,page.php
, etc.). - Custom widgets and sidebars.
- Custom post types and taxonomies.
- Theme customization options via the WordPress Customizer.
Conclusion
Building a WordPress theme from scratch can seem challenging, but by following these steps, you’ve created a basic yet functional theme. As you continue learning, you can add more features and customization to make your theme even better.
This guide provides a step-by-step approach for creating a simple WordPress theme.