Here’s a step-by-step guide on Creating a WordPress Plugin
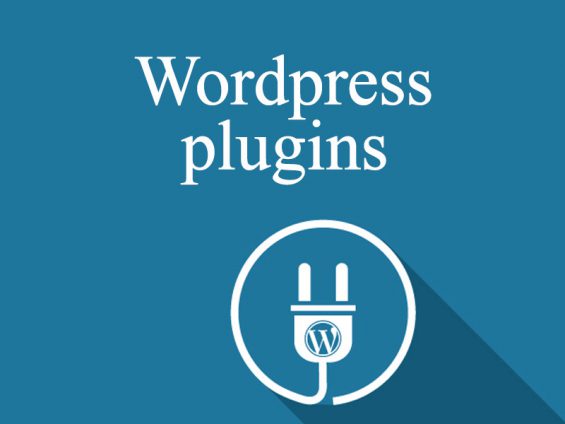
1. Preparation and Planning
Before you start developing your plugin, it’s important to define its purpose and requirements. Plan what your plugin will do: whether it will enhance functionality, add new content types, or integrate with external services.
2. Create a Plugin Folder
To start, create a folder for your plugin in the WordPress directory:
- Navigate to
wp-content/plugins
. - Create a folder with a unique name for your plugin, for example,
my-first-plugin
.
3. Create the Main Plugin File
Inside the plugin folder, create the main file with a .php
extension. Name it, for example, my-first-plugin.php
. This will be the main file handling the plugin’s functionality.
At the top of the file, add the following code:
<?php
/*
Plugin Name: My First Plugin
Plugin URI: https://example.com/my-first-plugin
Description: A simple plugin to demonstrate WordPress plugin creation.
Version: 1.0
Author: Your Name
Author URI: https://example.com
License: GPL2
*/
This code tells WordPress that this is a plugin, and it provides metadata about the plugin, such as its name, description, author, and version.
4. Add Basic Functionality
Now, you can add some basic functionality. For example, let’s create a function that displays a message at the bottom of every page:
function my_first_plugin_footer_message() {
echo '<p style="text-align: center; font-size: 18px;">This is my first WordPress plugin!</p>';
}
add_action('wp_footer', 'my_first_plugin_footer_message');
This code adds a message to the footer of every page when the plugin is activated. The add_action
function hooks our function into the wp_footer
action, which renders content at the bottom of each page.
5. Activation and Deactivation Hooks
To perform tasks upon activation or deactivation of the plugin, use the register_activation_hook
and register_deactivation_hook
. For example:
function my_first_plugin_activate() {
// Code to run when the plugin is activated
error_log('My First Plugin Activated');
}
function my_first_plugin_deactivate() {
// Code to run when the plugin is deactivated
error_log('My First Plugin Deactivated');
}
register_activation_hook(__FILE__, 'my_first_plugin_activate');
register_deactivation_hook(__FILE__, 'my_first_plugin_deactivate');
This code logs messages to the error log when the plugin is activated or deactivated.
6. Create an Admin Interface
If your plugin needs an admin interface (e.g., settings), you can add it using the admin_menu
hook to add a menu item in the WordPress admin panel.
function my_first_plugin_menu() {
add_menu_page(
'My First Plugin Settings', // Page title
'My First Plugin', // Menu title
'manage_options', // Capability
'my-first-plugin', // Unique identifier
'my_first_plugin_settings_page' // Function to display the page
);
}
function my_first_plugin_settings_page() {
echo '<div class="wrap">';
echo '<h1>My First Plugin Settings</h1>';
echo '<p>Settings for My First Plugin.</p>';
echo '</div>';
}
add_action('admin_menu', 'my_first_plugin_menu');
This code adds a new menu item in the admin panel called “My First Plugin,” which links to a simple settings page.
7. Test Your Plugin
After writing the plugin code, it’s important to test it on your WordPress site.
- Go to the WordPress admin panel.
- Navigate to the Plugins section and activate your plugin.
- Check that the plugin works properly (e.g., the footer message appears or the admin menu item shows up).
8. Optimization and Enhancements
Once the plugin is working, you can add additional features, such as:
- Creating settings — Add forms and fields to configure the plugin in the admin panel.
- Using hooks and filters — Extend functionality by using WordPress hooks and filters.
- Following coding standards — Keep your code clean and follow WordPress coding standards.
9. Distribute the Plugin
If you want to share your plugin with others, you can:
- Publish the plugin on the WordPress.org Plugin Repository.
- Create documentation for users.
- Add a description, screenshots, and installation instructions.
Conclusion
Creating a WordPress plugin is a fun process that allows you to add new functionality and features to your site. By understanding the basic plugin structure and utilizing the WordPress API, you can create more complex and useful solutions for WordPress websites.
With each new plugin, you’ll improve your skills and be able to create more sophisticated and feature-rich plugins.
This process will help you create your first plugin, but you can always enhance and expand its functionality as needed.